School Projects
Under construction! - projects are being uploaded
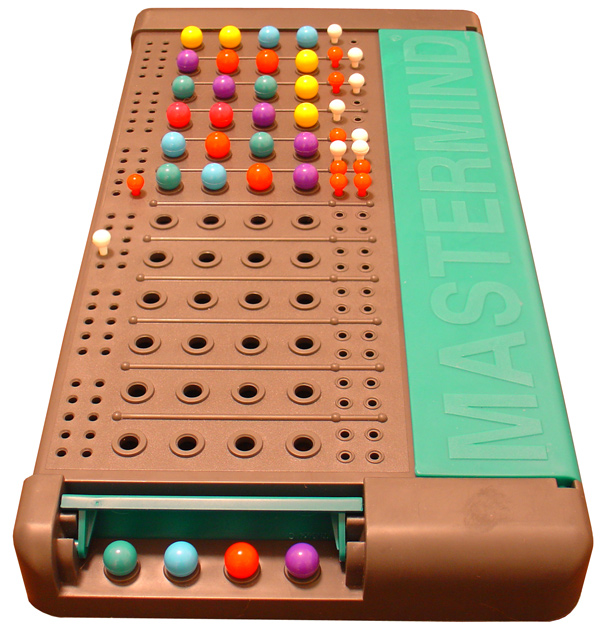
Mastermind Game - Visit
Background:
This project for completed for Intro to C class. It is the mastermind game in C. We completed it as one long project over the semester.
Process:
Over the course of the semester, we would add a new feature to the project weekly. It was really fun to complete as it featured so many relavant programming concepts. It was challenging, particularly using the C language, which tends to be much less forgiving than modern languages. Nonetheless, I am happy of the result.
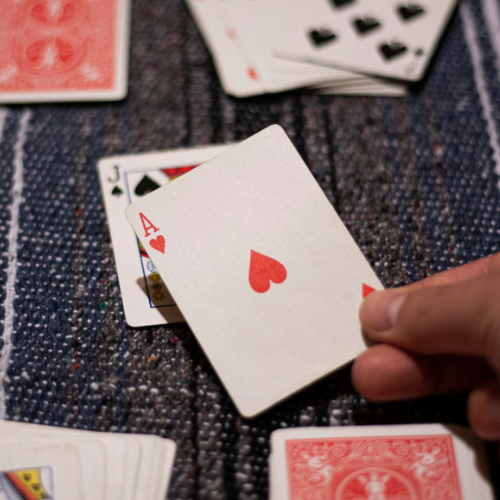
War Game - Visit
Background:
During our data structure curriculum on linked lists, we developed a game that simulates the classic card game "War." In this game, each card is represented as a node in a linked list, complete with its associated attributes such as suit and color.
Process:
The game "War" is played between two players, each with their own deck represented by a linked list. To run the game, I used a for loop to simulate five rounds, calling the playRound function during each iteration to determine the winner. This function acts as the driver for the game logic and is responsible for maintaining the linked list appropriately. One of the most challenging aspects of the project was implementing tie breakers in the game logic. Despite this difficulty, the project proved to be a fun and rewarding exercise in applying data structures to implement a game.
Binary Search Tree Arithmetic - Visit
Background:
As part of my study on elementary data structures, I completed a project on binary search trees that involved performing basic arithmetic operations on each node of two binary search trees.
Process:
To complete the project, I implemented a program that could traverse both binary search trees in an organized manner, performing arithmetic operations on corresponding nodes and creating a new binary search tree as the output. This required knowledge of various algorithms for tree traversal, as well as the ability to manipulate and update the binary search trees in an efficient way. Throughout the project, I gained valuable experience in data structures and algorithms, and developed my programming skills further.

Unfair Game - Visit
Background:
I completed a programming project called "The Unfair Game," which involved implementing the sorting algorithm quicksort to create an unbalanced game that was unfair to the player.
Process:
The objective of Team Captain A is to use this knowledge to choose the best players for their team and defeat Team Captain B's team.The selection process is to be done by both team captains, and the algorithm used for the selection process is not specified, but the prompt mentions that there is a program that uses quick-sort. The project required a deep understanding of the quick-sort algorithm and its implementation. Through this project, I gained valuable experience in algorithm design and implementation.
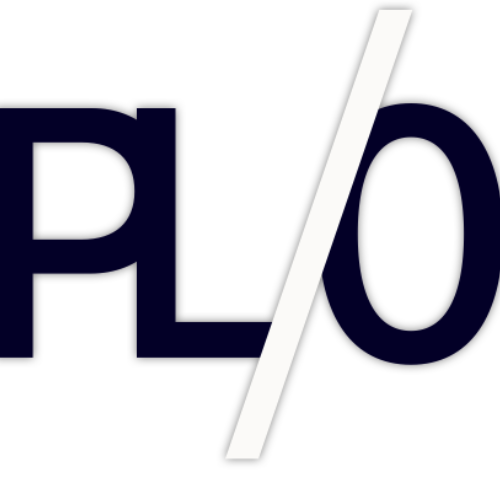
PL/0 Compiler - Visit
Background:
As part of my system software curriculum, I undertook a semester-long project to develop a compiler for the programming language PL/0. This involved designing and implementing a compiler that could translate PL/0 source code into machine-readable code, enabling the program to be executed by a computer.
Process:
The project was divided into three primary components, namely the lexical analyzer, the compiler/code generator, and the virtual machine. The project required a thorough understanding of the language's syntax and semantics, as well as knowledge of the tools and techniques used in compiler construction. Through this project, I gained valuable experience in software development and deepened my understanding of systems programming.

Java-Banking-Simulator - Visit
Background:
In my enterprise computing class, I worked on a project that underscored the importance of multithreading and safe access to shared locations. The project involved developing an application that utilized multiple threads to access a shared resource and process data concurrently.
Process:
To ensure the safety and integrity of the data, I implemented advanced techniques such as thread synchronization, and lock to ensure that all threads accessed the shared resource in a safe and orderly manner. These techniques prevented data corruption, race conditions, and other issues that could arise when multiple threads attempt to access the same resource simultaneously.The project also helped me to understand the importance of efficient resource utilization and the need to minimize resource contention. Overall, this project was a valuable learning experience that deepened my understanding of multithreading and safe access to shared locations.
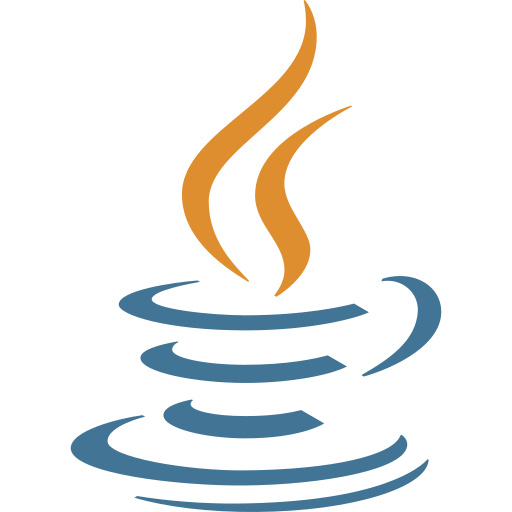
NileDotCom - Visit
Background:
In my enterprise computing class, I created a standalone GUI application that simulated an e-store. The program allowed users to add in-stock items, which were retrieved from a text file, to a shopping cart. Once all items were selected, the program totaled all costs, including tax, and produced an invoice. The program also appended a transaction log file for future reference.
Process:
To create the application, I utilized the Java swing library and incorporated a user-friendly graphical user interface (GUI) to make the program more intuitive and accessible to users. I also implemented error handling and input validation to ensure the program's robustness and prevent any unexpected errors from occurring. During the development process, I learned the importance of efficient file management and data retrieval, as well as the significance of providing users with clear and concise feedback. Additionally, I gained experience in GUI development.
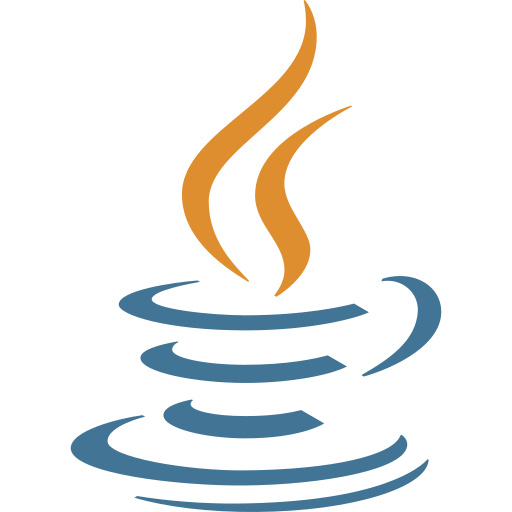
Client-Server-MySQL-App - Visit
Background:
In my enterprise computing class, I developed a two-tier client-server application utilizing MySQL and JDBC. The application features a Java GUI that allows users to interact with a secure and controlled database.
Process:
To ensure data security and access control, I implemented three distinct user roles. The root user was granted complete database control privileges, while the client user was limited to select commands. An operationslog user maintained a real-time log of all activity associated with the client application, including the number of queries and updates made.The project was designed with a specific focus on the utilization of JDBC, a powerful and flexible API for developing Java-based applications that interact with databases. Additionally, I gained experience with MySQL. This project provided an excellent opportunity to explore and experiment with the concepts of database management and application development.
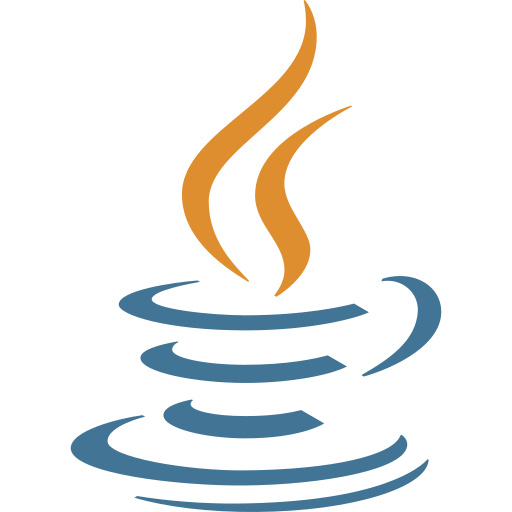
Three-Tier-Java-Web-App - Visit
Background:
Finally, as a capstone for my enterprise computing class,I developed a distributed three-tier web-based application that utilized Servlets and JavaServer Pages (JSP) technology, running on a Tomcat container/server. The application maintained a persistent MySQL database using JDBC, and was designed to enable secure and efficient database access and management.
Process:
To ensure proper access control, I implemented authentication that required users to provide valid login credentials before gaining access to the system. This was to prevent unauthorized access. To further enhance security, I implemented three distinct user roles. The client user role was limited to select commands, while the root user role had complete control privileges. The data entry user role could only perform insert commands. This approach helped to ensure the integrity of the database and minimize the risk of unauthorized access or data modification. I utilized Servlets and JSP technology, which provided a flexible and powerful framework for developing scalable, web-based applications. Overall, this project provided an excellent opportunity to explore and experiment with the concepts of web-based application development, distributed systems, and database management.